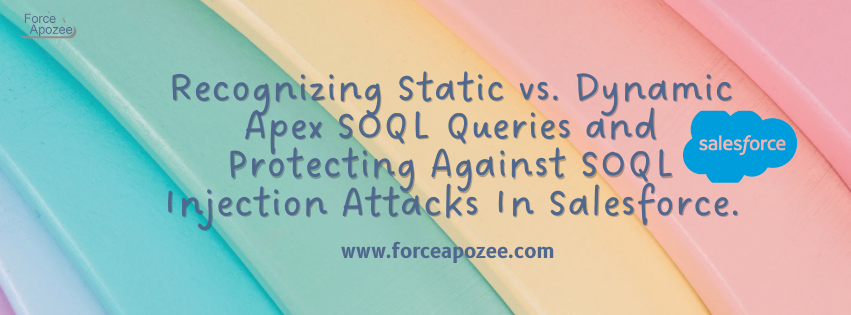
Introduction:
Comprehending the distinctions between static and dynamic SOQL (Salesforce Object Query Language) queries is essential when working with Salesforce Apex in order to create secure and effective applications. Both have their applications, but there are also concerns associated with them, particularly with regard to security flaws like SOQL injection attacks. Let's examine the main distinctions and recommended approaches for safeguarding your code against intrusions.
Static SOQL Queries:
Definition: Static SOQL queries are written in your Apex code directly, and compile time determines the query's structure. These queries are runtime constants since they are defined in the code as string literals.
For Example:
List<Account> accounts = [SELECT Company, Id FROM Account WHERE Sector = Technology'];
Benefits
Compile-Time Checks: Salesforce verifies the query for syntax mistakes and field-level security (FLS) violations during compile time because it is defined at that point.
Efficiency: Salesforce optimizes static queries during compilation, which results in a faster and more effective query.
Safety: Because static SOQL queries do not accept dynamic user input, they are intrinsically safe from SOQL injection attacks.
Use Cases:
When there is no need for runtime query structure modification because it is fixed.
When security and performance come first.
Dynamic SOQL Queries:
Definition: As dynamic SOQL queries are created as strings during runtime, they possess flexibility and can adjust to changes in user input or other variables.
For Example:
String industry = 'Technology';
String soql = 'SELECT Id, Name FROM Account WHERE Industry = \'' + industry + '\'';
List<Account> accounts = Database.query(soql);
Benefits
Flexibility: Dynamic SOQL is perfect for situations where query criteria must be decided dynamically since it lets you construct queries based on runtime
conditions.
Complex Queries: Allows for the creation of intricate queries that static SOQL might not be able to handle.
Drawbacks:
SOQL Injection Risk: If user input is directly entered into the query string without sufficient treatment, dynamic SOQL is susceptible to SOQL injection attacks.
Absence of Compile-Time Checks: The query is not compiled-time verified for mistakes or security flaws because it is created during runtime.
Use Cases:
When there are dynamic conditions, such as user input, that require the query structure to change.
When working with sophisticated sorting or filtering logic that is not specified.
Tips to Respond to SOQL Injection Operations:
When a user enters a query incorrectly, it's known as SOQL injection. This might lead to unexpected outcomes or allow an attacker to access data that is not authorized. Employing dynamic SOQL searches carries a substantial risk.
The best ways to stop SOQL injection are as follows:
1.Use bind variables: The best defense against SOQL injection is the use of bind variables. By leaving the input escaping to Salesforce, they let you securely include user input into dynamic queries.
String industry = 'Technology';
List<Account> accounts = [SELECT Id, Name FROM Account WHERE Industry = :industry];
2.Escape Single Quotes: When concatenating strings, make sure to escape single quotes to stop malicious code from being injected by attackers.
3.Apply Whitelisting: Assign a set of permissible values to each input and discard any that deviates from the anticipated pattern.
Set<String> allowedIndustries = new Set<String>{'Technology', 'Finance', 'Healthcare'};
if (allowedIndustries.contains(industry)) {
String soql = 'SELECT Id, Name FROM Account WHERE Industry = \'' + industry + '\'';
List<Account> accounts = Database.query(soql);
} else {
// Handle invalid input
}
4.Avoid Dynamic SOQL Where Possible: To completely eliminate the risk, utilize static SOQL queries whenever feasible. If dynamic SOQL is required, carefully adhere to the guidelines above.
Conculsion:
Salesforce developers must comprehend the distinctions between static and dynamic SOQL queries. Dynamic SOQL delivers the flexibility required for more complicated applications, while static SOQL offers compile-time safety and performance benefits. But this flexibility also means that you have to take precautions to keep your application safe from SOQL injection attempts. You can protect your code against these vulnerabilities by adhering to recommended practices including using bound variables, escaping user input, and putting whitelisting in place.
Put these tactics into practice to make sure your Salesforce apps are safe and effective. Have fun with coding!
Knowledgeable blog