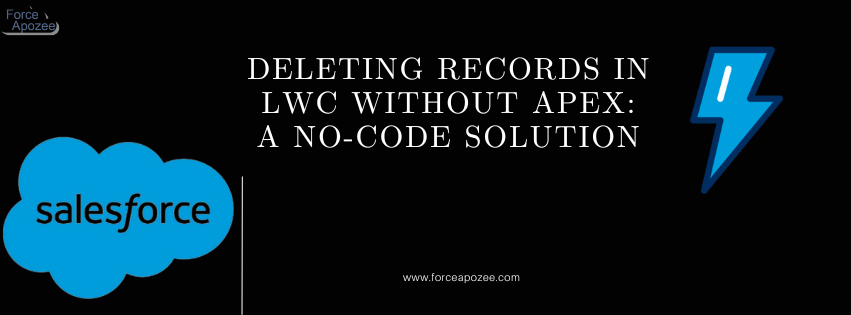
To delete records in Lightning Web Components (LWC) without using Apex, you can leverage the deleteRecord method from the lightning/uiRecordApi module. This approach allows you to Deleting a record directly in LWC using Salesforce's Lightning Data Service (LDS) without the need for a custom Apex controller Apex: A No-CodeSolution.
Here's how you can implement it:
Deleting Records in LWC Without Apex: A No-CodeSolution Steps:
Import the necessary modules: Import deleteRecord from lightning/uiRecordApi and ShowToastEvent from lightning/platformShowToastEvent.
Call the deleteRecord method: Use the deleteRecord method to delete the record by passing the record ID.
Handle Success or Error: Show a toast message for success or error to give feedback to the user.
import { LightningElement, api } from 'lwc';
import { deleteRecord } from 'lightning/uiRecordApi';
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
export default class DeleteRecordLWC extends LightningElement {
@api recordId; // Record ID to delete (this can be passed from the parent component or fetched dynamically)
handleDelete() {
deleteRecord(this.recordId)
.then(() => {
this.dispatchEvent(
new ShowToastEvent({
title: 'Success',
message: 'Record deleted successfully',
variant: 'success',
}),
);
// Additional logic after record deletion (e.g., navigation, refresh data, etc.)
})
.catch(error => {
this.dispatchEvent(
new ShowToastEvent({
title: 'Error deleting record',
message: error.body.message,
variant: 'error',
}),
);
});
}
}
Key Points:
@api recordId: This decorator allows the component to accept a record ID from outside, such as a parent component.
deleteRecord(this.recordId): This deletes the record with the specified recordId.
Error handling: It uses .catch to handle any errors that may occur during the deletion process.
Use Cases:
You can use this method when you need to delete a record in a single record context (e.g., within a detail view or in a row of a datatable).
It simplifies the deletion process as it avoids writing Apex and uses standard Salesforce APIs.
Conculsion
Deleting records in LWC without Apex simplifies development by leveraging the deleteRecord method from lightning/uiRecordApi, providing efficient, declarative control within Lightning Data Service.
Comments